Any property with a Delegate type can store an Action Graph.
public class ExampleComponent : Component
{
[Property]
public Action DoSomething { get; set; }
}attribute 'data-code' not allowedattribute 'data-language' not allowedattribute 'contenteditable' not allowedattribute 'blocktype' not allowed

attribute 'contenteditable' not allowedattribute 'blocktype' not allowedattribute 'data-image-style' not allowed
Action is the simplest, but you can have parameters too.
[Property]
public Action<int, GameObject, string, object[]> DoSomethingElse { get; set; }attribute 'data-code' not allowedattribute 'data-language' not allowedattribute 'contenteditable' not allowedattribute 'blocktype' not allowed
attribute 'contenteditable' not allowedattribute 'blocktype' not allowed
You can invoke a graph like any other method.
protected override void OnStart()
{
DoSomething();
DoSomethingElse( 123, GameObject.Parent, "Hello", new object[10] );
}attribute 'data-code' not allowedattribute 'data-language' not allowedattribute 'contenteditable' not allowedattribute 'blocktype' not allowed
Finally, you can make your own nodes in C# as static methods.
//
// Increments the value by 1.
//
[ActionGraphNode( "example.addone" ), Pure]
[Title( "Add One" ), Group( "Examples" ), Icon( "exposure_plus_1" )]
public static int AddOne( int value )
{
return value + 1;
}
//
// Waits for one second.
//
[ActionGraphNode( "example.waitone" )]
[Title( "Wait One" ), Group( "Examples" ), Icon( "hourglass_bottom" )]
public static async Task WaitOne()
{
await Task.Delay( TimeSpan.FromSeconds( 1d ) );
}attribute 'data-code' not allowedattribute 'data-language' not allowedattribute 'contenteditable' not allowedattribute 'blocktype' not allowed
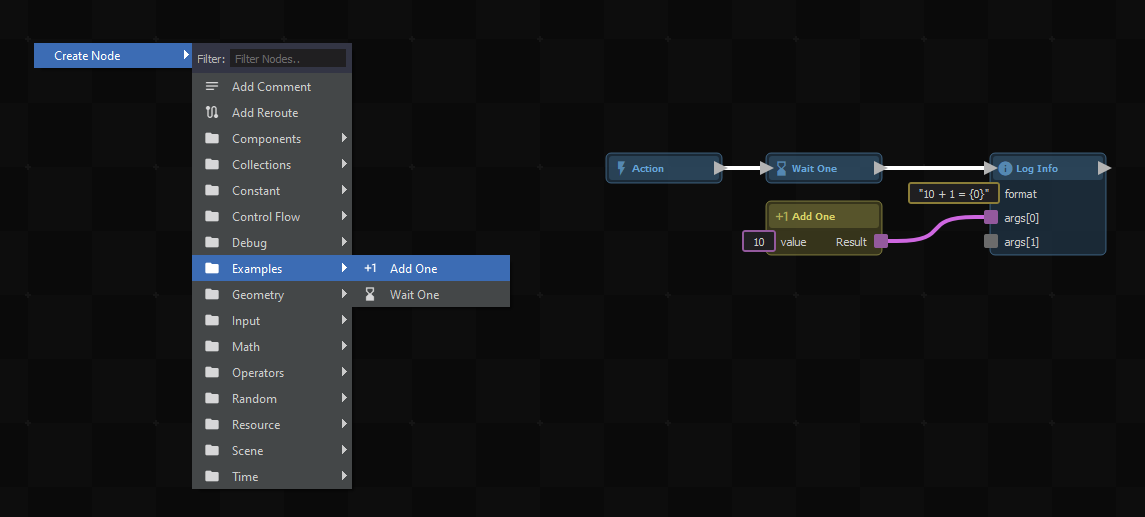
attribute 'contenteditable' not allowedattribute 'blocktype' not allowed
This seems vastly more complicated, confusing, and time consuming compared to what thousands of mappers have been using for decades. :(